How to use the BBC Micro Bit accelerometer
Orienteering
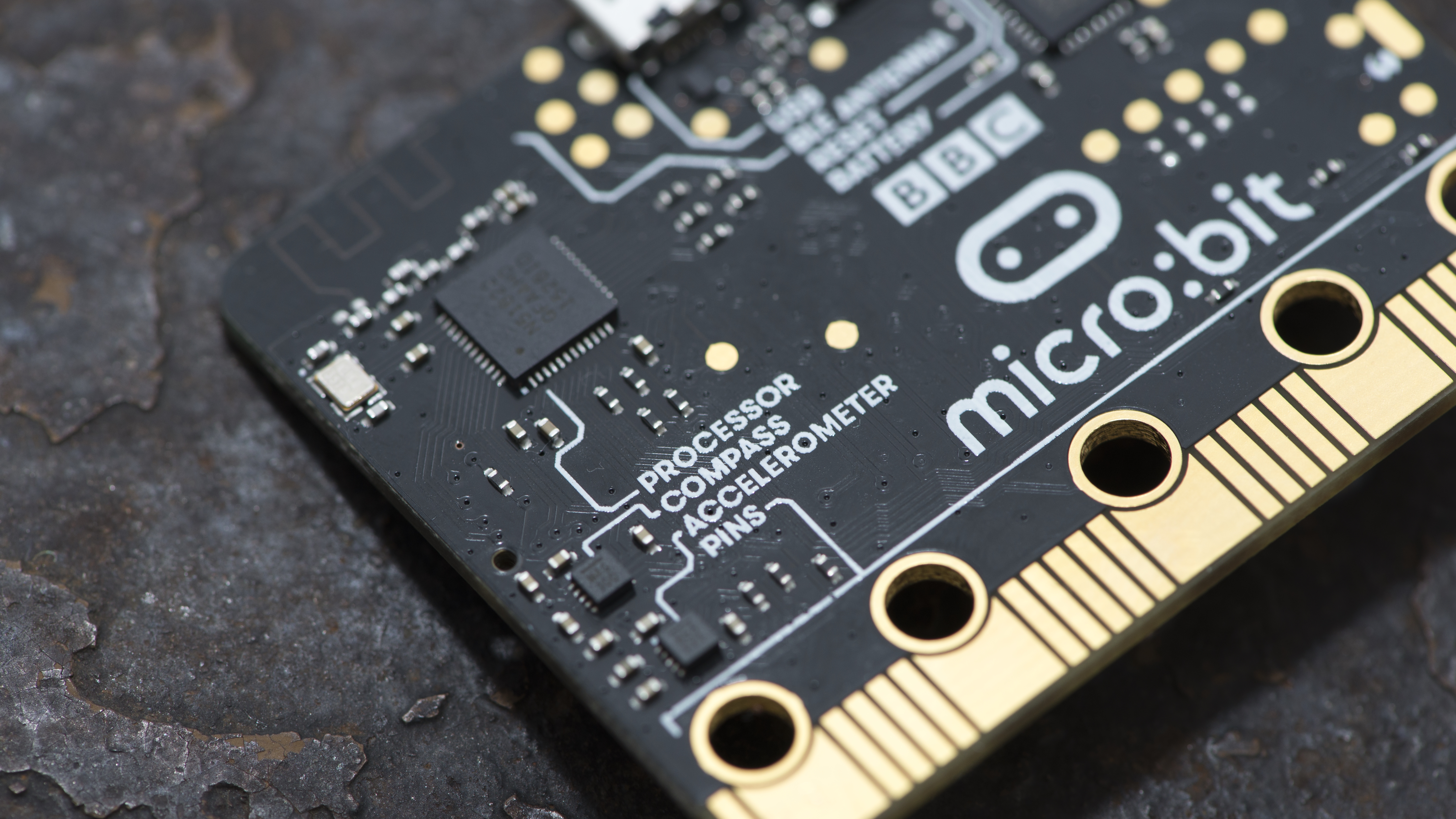
For this project you'll just need a BBC micro:bit, as this comes with an accelerometer, commonly used in mobile devices to determine device orientation and screen rotation. In this tutorial we shall use the micro:bit as an input device that reacts to gestures.
It's worth checking out our how to get started with the BBC Micro Bit guide if you haven't read that before you start on this project.
We begin in the Mu editor and as always our first line imports the micro:bit library:
from microbit import *
Now we use an infinite loop to contain the code that we wish to run while True.
The accelerometer embedded in the micro:bit has its own series of functions that can be used to query the position of the board in space. We can detect the full x,y and z co-ordinates of the board for fine control but there are times where we do not need such precision, and that is where gestures provide a quick solution.
Gestures are motions that have been predetermined, for example shaking, tilting and flipping the micro:bit. We can use these gestures for simple input and in this project we're going to use a conditional statement that will check to see which gesture has been made and react accordingly.
Sign up for breaking news, reviews, opinion, top tech deals, and more.
The first test is to see if the accelerometer has been tilted upwards. The output from this test is either True or False, but if True then the code indented below is activated. Clearing the screen before scrolling text across the micro:bit LED matrix. Lastly there is a 0.1 second pause before the code checks again.
First, type
if accelerometer.was_gesture('up'):
Then type the following, making sure each line is indented by pressing the Tab key on your keyboard.
display.clear()
display.scroll("Dogs cannot look up")
sleep(100)
Our next test is called "Else If.." abbreviated in the Python coding language to "elif". In this test we check to see if the micro:bit has been pointed towards the floor. First type:
elif accelerometer.was_gesture('down'):
Then again make each following line indented using the Tab key.
display.clear()
display.scroll("I feel sick")
sleep(100)
We repeat this process for two more tests that will cover tipping the micro:bit left and right. The syntax for this code is identical to the down gesture, but refers to 'left' and 'right'.
Our last gesture is a shaking motion, which when detected will trigger the final section of code to be activated. Write:
elif accelerometer.was_gesture('shake'):
Then underneath, again with each line indented:
display.clear()
display.scroll("Stop shaking me!")
sleep(100)
With the code now complete, save your work and then click on Flash to flash the code on to the attached micro:bit. After the yellow LED on the reverse of the micro:bit stops flashing you are ready to use the controller.
Start by tipping the micro:bit forwards, backwards and side to side. Last, shake the micro:bit to test the shake gesture.
- Enjoyed this article? Expand your knowledge of Linux, get more from your code, and discover the latest open source developments inside Linux Format. Read our sampler today and take advantage of the offer inside.