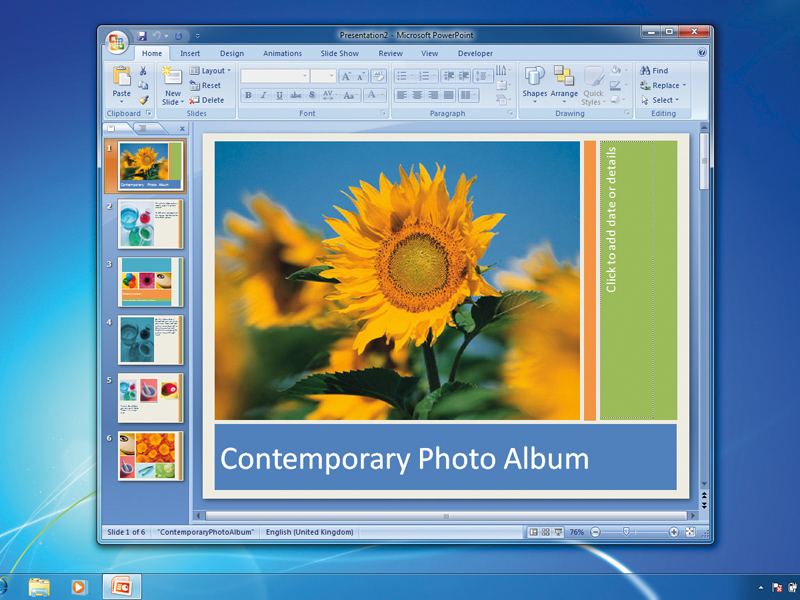
The first part of this tutorial explained how AutoIt can help you to automate tasks by simulating keypresses. But while this is a powerful technique that enables you to control just about any application, it does have its problems.
The scripting code quickly becomes cryptic; there's no way to tell what a keypress sequence does without looking at the app. It's not always reliable, either – a simple interface change can break your keypress sequence and force you to start again.
However, there's a smarter automation alternative out there called the Component Object Model (COM). Some apps use it to make their functionality available to other scripts and programs, including Windows, Microsoft Office, Skype and many others. Using it you can send emails in Outlook, automate Skype calls, create spreadsheets and more.
Getting started
The first step in using COM is to create a variable that refers to the application object we need (or create an instance of the object, in programmer-speak). In AutoIt, that looks something like this:
$oShell = ObjCreate("Shell. Application")
The 'sign tells us that this is a variable called '$oShell'. We use a lower-case 'o' as the first letter of the variable name to reminds us that this is an object. We then ask the ObjCreate function to create a 'Shell.Application' object. This is a copy of Explorer that gives us instant access to lots of useful Windows features.
Sign up for breaking news, reviews, opinion, top tech deals, and more.
The easiest way to make use of an object is to apply one of its methods. These are commands that the object makes available. Our Shell.Application object, for instance, has a method called 'MinimizeAll' that will minimise all open application windows so we can see the desktop. We can use it by applying that method name to the variable we've just created, like this:
$oShell. MinimizeAll.
Similarly, we can use $oShell.UndoMinimizeAll to undo the minimise command, restoring application windows to their previous positions. We can also rearrange windows by appending TileHorizontally, TileVertically or alternatively CascadeWindows.
Our Shell.Application object provides plenty of handy shortcuts, too. If we want to launch the Search window, for instance, then we can use this:
$oShell.FindFiles.
Then there's the 'FileRun' method, which launches the Run box; 'SetTime', which opens the Date and Time dialog; and 'ControlPanelItem', which displays an applet that you specify. For example, typing ControlPanel Item("Inetcpl.cpl") will launch the Internet Options dialog.
Need some more? Check out the official Microsoft Shell.Application documentation for the full list of methods here.
Controlling services
We've started gently with some of the simpler Shell.Application options, but the object provides plenty of more interesting features for us to explore.
The 'AddToRecent' method, for instance, will add the document you specify to the Windows Recent Files list. Use it like this (ensuring that 'c:\file.ext' is replaced with the path of a real file on your PC):
$oShell = ObjCreate(FShell.Application") $oShell.AddToRecent("c:\file.ext")
By adding more file names, you could customise a script like this to ensure that the Recent Files list always includes a particular set of commonly used documents.
Alternatively, you could protect your privacy by creating a script that replaces the Recent Files list with your choice of files, so nobody can use it to keep an eye on what you've been doing. The ability to stop and start Windows services introduces more interesting possibilities.
You could create a script to close down non-essential services to save resources before you run a demanding game, for instance, and another that will start them again afterwards. Here are a couple of short examples of this:
StopServices.au3
$oShell = ObjCreate("Shell. Application")
$oShell.ServiceStop("wuaserv", false)
$oShell.ServiceStop("wsearch", false)
StartServices.au3
$oShell = ObjCreate("Shell. Application")
$oShell.ServiceStart("wuaserv", false)
$oShell.ServiceStart("wsearch", false)
Here the 'StopServices.au3' script begins by creating a Shell.Application object, then uses the 'ServiceStop' method to stop the 'wuaserv' (Windows Update) and 'wsearch' (Windows Search) services. The second ServiceStop value tells Windows whether you want this change to be permanent. Set it to 'true' and Windows will disable the service, so it won't start when you next reboot.
However, as the Search and Windows Update services are important, we only want to turn them off temporarily, so we should set the second value to false. Run this script first to free up RAM and system resources, then launch your hungry app. When you're done, run the StartServices script and it'll reverse the process, using the ServiceStart method to relaunch services that were closed.
If you forget to run StartServices, don't worry – any services you've paused will be started again the next time you reboot. To make this really useful we could expand it to include other non-essential services. Candidates for this include Spooler (the print spooler), BITS (Background Intelligent Transfer Service), EMDMgmt (ReadyBoost) and third-party services such as Apple Mobile Device (only necessary when you're connecting an iPod or iPhone to your PC).
To explore this further, run services.msc to view the services that are active on your PC. Find a service that you think is nonessential and could be turned off safely for a while. Double-click it and note the service name. Disabling a critical service could disable your PC, so check at www.blackviper.com to confirm that a service is safe to turn off temporarily.
If it is, add $oShell.ServiceStop("W32Time", false) to StopServices.au3 and $oShell. ServiceStart("W32Time", false) to StartServices.au3.